You should create a free 100Pay account which will give you access to your private and public API keys.
We're going to use our public keys for this demo. To get your public key, login into your 100Pay dashboard and click on the Settings tab, and then click on Developers Settings.
You'll notice you have two keys: Public and Private. While building your app, you'll need the API keys to access the APIs.
Since this is a client-side integration, it means that our API keys will be exposed. To prevent anyone gaining access to our 100Pay account, we want to make sure we're using our Public keys
. Private keys
should only ever be used on the server.
So, let's get to coding! To start off, we'll create a new Vue app.
# For Yarn Modern (v2+)
yarn create vue@latest
# For Yarn ^v4.11
yarn dlx create-vue@latest
Once our app is created, we'll need to navigate into our app's directory and start our app:
cd vue-project
npm install
Let's take a moment and add the UI for our checkout page.
<template>
<form>
<div className="wrapper">
<!-- name -->
<div className="input-group">
<label htmlFor="name">Name</label>
<input type="text" id="name" name="name" />
</div>
<!-- email -->
<div className="input-group">
<label htmlFor="email">Email</label>
<input type="email" id="email" name="email" />
</div>
<!-- phone -->
<div className="input-group">
<label htmlFor="phone">Phone</label>
<input type="tel" id="phone" name="phone" />
</div>
<!-- currency -->
<div className="input-group">
<label htmlFor="currency">Currency</label>
<select name="currency" id="currency">
<option value="usd">USD</option>
<option value="eur">EUR</option>
<option value="gbp">GBP</option>
</select>
</div>
<!-- amount -->
<div className="input-group">
<label htmlFor="amount">Amount</label>
<input type="number" id="amount" name="amount" />
</div>
<!-- submit -->
<div className="action-cont">
<button type="submit">Submit</button>
</div>
</div>
</form>
</template>
Now you should be able to see our beautiful form display in your browser! Use the form to capture user details and payment information. The form includes fields for name, email, phone, currency, and amount.
This will help streamline the checkout process on your site. Ensure all fields are correctly filled out to facilitate smooth transactions through the 100Pay Checkout API.
But our form isn't functional yet. We need to add some logic that will submit our customer's data and initialize a transaction on 100Pay. Let's install the 100Pay Checkout SDK:
npm install @100pay-hq/checkout
pnpm add @100pay-hq/checkout
yarn add @100pay-hq/checkout
<script src="https://js.100pay.co/"></script>
Once the library installs successfully, we can add some variables to hold state and a function to handle the state changes. For now we'll just hardcode our publicKey since it won't be changing.
<script setup>
import {ref} from "vue";
const PUBLIC_KEY = "your_pk_here";
const name = ref("");
const email = ref("");
const phone = ref("");
const currency = ref("USD");
const ammount = ref(0);
const handleSubmit = (e) => {
e.preventDefault();
console.log("Submitting")
}
</script>
<template>
<form @submit="handleSubmit">
<div className="wrapper">
<!-- name -->
<div className="input-group">
<label htmlFor="name">Name</label>
<input v-model="name" type="text" id="name" name="name" required />
</div>
<!-- email -->
<div className="input-group">
<label htmlFor="email">Email</label>
<input v-model="email" type="email" id="email" name="email" required />
</div>
<!-- phone -->
<div className="input-group">
<label htmlFor="phone">Phone</label>
<input v-model="phone" type="tel" id="phone" name="phone" required />
</div>
<!-- currency -->
<div className="input-group">
<label htmlFor="currency">Currency</label>
<select v-model="currency" name="currency" id="currency" required>
<option value="USD">USD</option>
<option value="EUR">EUR</option>
<option value="GBP">GBP</option>
</select>
</div>
<!-- amount -->
<div className="input-group">
<label htmlFor="amount">Amount</label>
<input
v-model="amount"
type="number"
id="amount"
name="amount"
min="0.1"
step="0.1"
required
/>
</div>
<!-- submit -->
<div className="action-cont">
<button type="submit">Submit</button>
</div>
</div>
</form>
</template>
After completing the form and states for the form, import shop100Pay
and call the checkout function to initiate the payment process. The shop100Pay
function is the main entry point for integrating 100Pay's checkout process into your application. We will call it payWith100Pay
.
<script setup lang="ts">
import { ref } from 'vue'
import { shop100Pay } from '@100pay-hq/checkout'
type PayWith100PayType = {
name: string
email: string
phone: string
currency: string
amount: number
publicKey: string
}
const PUBLIC_KEY =
'LIVE;PK;eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJhcHBJZCI6IjY1ZjljZjVhZTZjNTdlMDAyZDc5NmM1NiIsInVzZXJJZCI6IjY1ZjljZjVhZTZjNTdlMDAyZDc5NmM1MiIsImlhdCI6MTcxMDg3MDM2Mn0.l-XuOcbxZWBdRAVt5Mzz1v97uRSB40K3xrrGaSHg4N0'
const name = ref('')
const email = ref('')
const phone = ref('')
const currency = ref('USD')
const amount = ref(0)
const payWith100Pay = ({ name, email, phone, currency, amount, publicKey }: PayWith100PayType) => {
shop100Pay.setup({
ref_id: '' + Math.floor(Math.random() * 1000000000 + 1),
api_key: publicKey,
customer: {
user_id: '1', // optional
name: name,
phone,
email
},
billing: {
amount: amount,
currency: currency, // or any other currency supported by 100pay
description: 'Test Payment',
country: 'US',
vat: 10, //optional
pricing_type: 'fixed_price' // or partial
},
metadata: {
is_approved: 'yes',
order_id: 'OR2', // optional
charge_ref: 'REF' // optionalm, you can add more fields
},
call_back_url: 'http://localhost:8000/verifyorder/',
onClose: () => {
alert('You just closed the crypto payment modal.')
},
onPayment: (reference) => {
console.log(`New Payment detected with reference ${reference}`)
},
onError: (error) => {
// handle your errors, mostly caused by a broken internet connection.
console.log(error)
alert('Sorry something went wrong please try again.')
},
callback: (response) => {
console.log(response)
}
})
}
const handleSubmit = (e: Event) => {
e.preventDefault()
console.log('Submitting')
// call the function to initialize the payment and show the popup
payWith100Pay({
name: name.value,
email: email.value,
phone: phone.value,
currency: currency.value,
amount: amount.value,
publicKey: PUBLIC_KEY
})
}
</script>
<template>
<form @submit="handleSubmit">
<div className="wrapper">
<!-- name -->
<div className="input-group">
<label htmlFor="name">Name</label>
<input v-model="name" type="text" id="name" name="name" required />
</div>
<!-- email -->
<div className="input-group">
<label htmlFor="email">Email</label>
<input v-model="email" type="email" id="email" name="email" required />
</div>
<!-- phone -->
<div className="input-group">
<label htmlFor="phone">Phone</label>
<input v-model="phone" type="tel" id="phone" name="phone" required />
</div>
<!-- currency -->
<div className="input-group">
<label htmlFor="currency">Currency</label>
<select v-model="currency" name="currency" id="currency" required>
<option value="USD">USD</option>
<option value="EUR">EUR</option>
<option value="GBP">GBP</option>
</select>
</div>
<!-- amount -->
<div className="input-group">
<label htmlFor="amount">Amount</label>
<input
v-model="amount"
type="number"
id="amount"
name="amount"
min="0.1"
step="0.1"
required
/>
</div>
<!-- submit -->
<div className="action-cont">
<button type="submit">Submit</button>
</div>
</div>
</form>
</template>
From the code above, we integrate the 100Pay SDK into the Vue app's checkout form. Here's a breakdown of how it works:
- State Management with
ref
:
We use the ref
function from Vue to manage reactive state for user input fields like name
, email
, phone
, currency
, and amount
. These refs
ensure that any changes in the form fields are tracked and updated in real-time. - 100Pay SDK Setup:
We import the
shop100Pay
function from the 100Pay SDK, which is used to initiate the payment process. This function is called within the payWith100Pay
function that we defined. - Payment Initialization:
The
payWith100Pay
function prepares the necessary parameters (like user information and payment details) and then calls shop100Pay.setup()
. This method configures the 100Pay payment modal and triggers it when the form is submitted.
Parameters:ref_id
: A randomly generated reference ID for the transaction.api_key
: The public API key obtained from the 100Pay dashboard.customer
: Information about the customer (name, email, and phone).billing
: Payment details such as the amount, currency, and description.metadata
: Additional optional information like order ID and charge reference.call_back_url
: The URL to redirect the user to after a successful payment.onClose
: A function to handle the modal closing event.onPayment
: A callback function to handle payment completion events.onError
: A function to handle any errors during the payment process.callback
: A general callback function to handle the response from 100Pay.
- Form Submission:
The
handleSubmit
function prevents the default form submission behavior and then calls payWith100Pay
, passing the user's input values (name, email, phone, currency, and amount) as well as the public API key. This initiates the payment process and opens the 100Pay payment modal.
Here's our app so far in action:
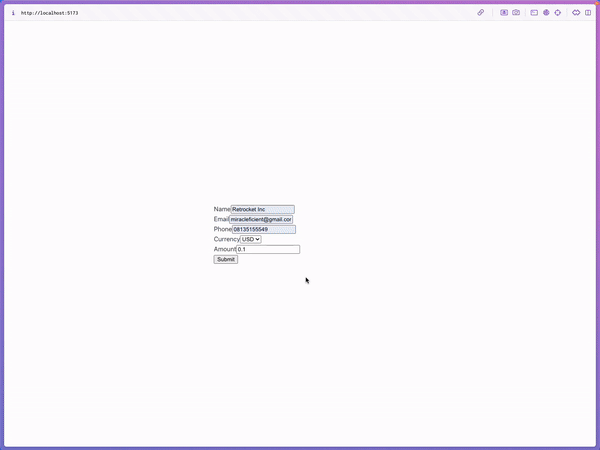
That’s it! You’ve successfully integrated 100Pay Checkout into your Vue application. This setup allows your customers to make payments with ease, and the 100Pay modal ensures that their payment details are securely handled.
In a real-world scenario, make sure to test the payment process thoroughly. Also, ensure that your backend securely verifies payments and updates your records accordingly.
By now, you've successfully integrated the 100Pay Checkout with your Vue application! Here's a quick recap of what we covered:
- Setting up a Vue project.
- Creating a checkout form with user input fields.
- Installing the 100Pay SDK.
- Handling state and form submission using Vue's
ref
and methods. - Initializing the payment process using the
shop100Pay
function from the 100Pay SDK.
Once the payment modal is triggered, users can complete the payment process with their chosen cryptocurrency, and you will be able to capture successful payments on your platform.
View the code for this example on GitHub